#구초제 포인터 정의
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
#include <iostream>
//구조체 포인터
struct ProductInfo {
int num;
char name[100];
int cost;
};
int main(){
ProductInfo myProduct = { 4797283, "제주 한라봉", 19900};
ProductInfo *ptr_product = &myProduct;
std::cout << "상품 번호 : " << (*ptr_product).num << std::endl;
std::cout << "상품 이름 : " << (*ptr_product).name << std::endl;
std::cout << "가 격 : " << (*ptr_product).cost << "원" << std::endl;
}
|
cs |
(*ptr_product). num
(*ptr_product). name
(*ptr_product). cost
이다.
여기서, (*ptr_product).num을 *ptr_product.num으로 쓴다면,
*ptr_product.num은 *(ptr_product.name)으로 인식하기에 오류가 발생한다.
따라서, 구조체 포인터는 (*ptr_product).num 와 같은 형식으로 써야 한다.
그렇지만, 하나하나 괄호를 사용하여 쓰기는 불편하다.
그래서, 쓸 수 있는 표현이 하나 더 있다.
- ->
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
#include <iostream>
//구조체 포인터
struct ProductInfo {
int num;
char name[100];
int cost;
};
int main(){
ProductInfo myProduct = { 4797283, "제주 한라봉", 19900};
ProductInfo *ptr_product = &myProduct;
std::cout << "상품 번호 : " << ptr_product->num << std::endl;
std::cout << "상품 이름 : " << ptr_product->name << std::endl;
std::cout << "가 격 : " << ptr_product->cost << "원" << std::endl;
}
|
cs |
위의 예시처럼, (*ptr_product).name과 ptr_product->name은 같은 표현이다.
#구조체에 함수 집어넣기
먼저, 구조체 밖에 함수를 정의하는 코드는,, 이런 식으로 짠다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
#include <iostream>
//구조체 포인터
struct ProductInfo {
int num;
char name[100];
int cost;
};
//percent만큼 할인하는 함수
void productSale(ProductInfo *p, int percent) { //call-by-reference
p->cost -= p->cost * percent / 100;
}
int main(){
ProductInfo myProduct = { 4797283, "제주 한라봉", 19900};
productSale(&myProduct, 10);
std::cout << "상품 번호 : " << myProduct.num << std::endl;
std::cout << "상품 이름 : " << myProduct.name << std::endl;
std::cout << "가 격 : " << myProduct.cost << "원" << std::endl;
}
|
cs |
구조체 밖에서 구조체를 사용하는 함수를 작성할 때는, 구조체 자체를 포인터를 사용해 함수로 넘겨준다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
#include <iostream>
//구조체 포인터
struct ProductInfo {
int num;
char name[100];
int cost;
};
//두 product를 바꾸는 함수
void productSwap(ProductInfo *a, ProductInfo *b){
ProductInfo tmp = *a;
*a = *b;
*b = tmp;
}
int main(){
ProductInfo myProduct = { 4797283, "제주 한라봉", 20000};
ProductInfo otherProduct = { 4797283, "성주 꿀참외", 10000};
productSwap(&myProduct, &otherProduct);
}
|
cs |
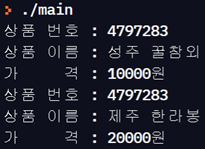
구조체 밖의 함수에서,
두 변수의 값을 서로 교환하듯이,
두 구조체의 값을 서로 교환하는 코드이다.
c++에서는 함수에 구조체를 넘길 때 이런 식으로도 가능하다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
#include <iostream>
//초(sec)를 계산하기
struct Time {
int h, m, s;
};
int totalSec(Time t){
return 3600*t.h + 60*t.m + t.s;
}
int main(){
Time t = {1,22,48};
std::cout << totalSec(t);
}
|
cs |
여기서 totalSec의 매개변수인 t와 main 안에 totalSec(t)의 t는 서로 다른 것
이렇게 위에서 보여준 구조체를 매개변수로 가진 함수는 구조체와 밀접한 관련이 있기 때문에,
함수를 구조체 안으로 넣어버리면, 더욱 편리해질 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
//초(sec)를 계산하기
struct Time {
int h, m, s;
//초 계산 함수
int totalSec(){
return 3600*h + 60*m + s;
}
};
int main(){
Time t = {1,22,48};
std::cout << t.totalSec();
}
|
cs |
위의 코드의 함수를 구초체 안으로 집어 넣으면, 이렇게 표현할 수 있다.
구조체 안에 있고, 함수에서는 구조체 안의 변수만을 사용하기 때문에,
함수를 호출할 땐, 어떠한 변수도 적을 필요가 없게 된다.
결과 값 또한 위의 코드와 똑같이 나온다.
구조체 안에 함수를 넣을 경우, 구조체의 멤버변수들을 일반 변수처럼 사용할 수 있고,
구조체의 함수를 구조체 밖에서 호출할 때, 다른 구조체의 멤버변수를 호출하는 것과 같은 방법으로 호출하면 된다.
출처(source) - 유튜브 두들낙서님
https://www.youtube.com/c/%EB%91%90%EB%93%A4%EB%82%99%EC%84%9C
두들낙서
C/C++ 강좌를 올리고 있고 다른 컨텐츠는 할 수도 있고 안 할 수도 있는 채널. ▶ 두들낙서 지식공유 서버 참가하기: https://discord.gg/y4SXcjU
www.youtube.com
c++ 최고의 강좌,,
'공부 > c++' 카테고리의 다른 글
#15 상수 만들기 | const | 매크로 | enum | c++ (0) | 2021.09.20 |
---|---|
#14 이중 포인터와 typedef (0) | 2021.09.20 |
#12 구조체 | struct | c++ (0) | 2021.09.20 |
#11 typedef (0) | 2021.09.20 |
#10 배열을 매개변수로 넘기기 문제 | 띄어쓰기없이 출력하기 (0) | 2021.09.20 |